Positional Patterns in C#: The What, Why, and How
C# has been evolving steadily, and with C# 8.0 and beyond, pattern matching got a serious power-up.
One of the coolest additions is Positional Patterns.
If you’ve ever written code that checks for multiple values inside a class or struct, this feature might just make your code cleaner and easier to read.
In this post, let me walk you through what positional patterns are, when to use them, and how they compare to other matching techniques like property patterns.
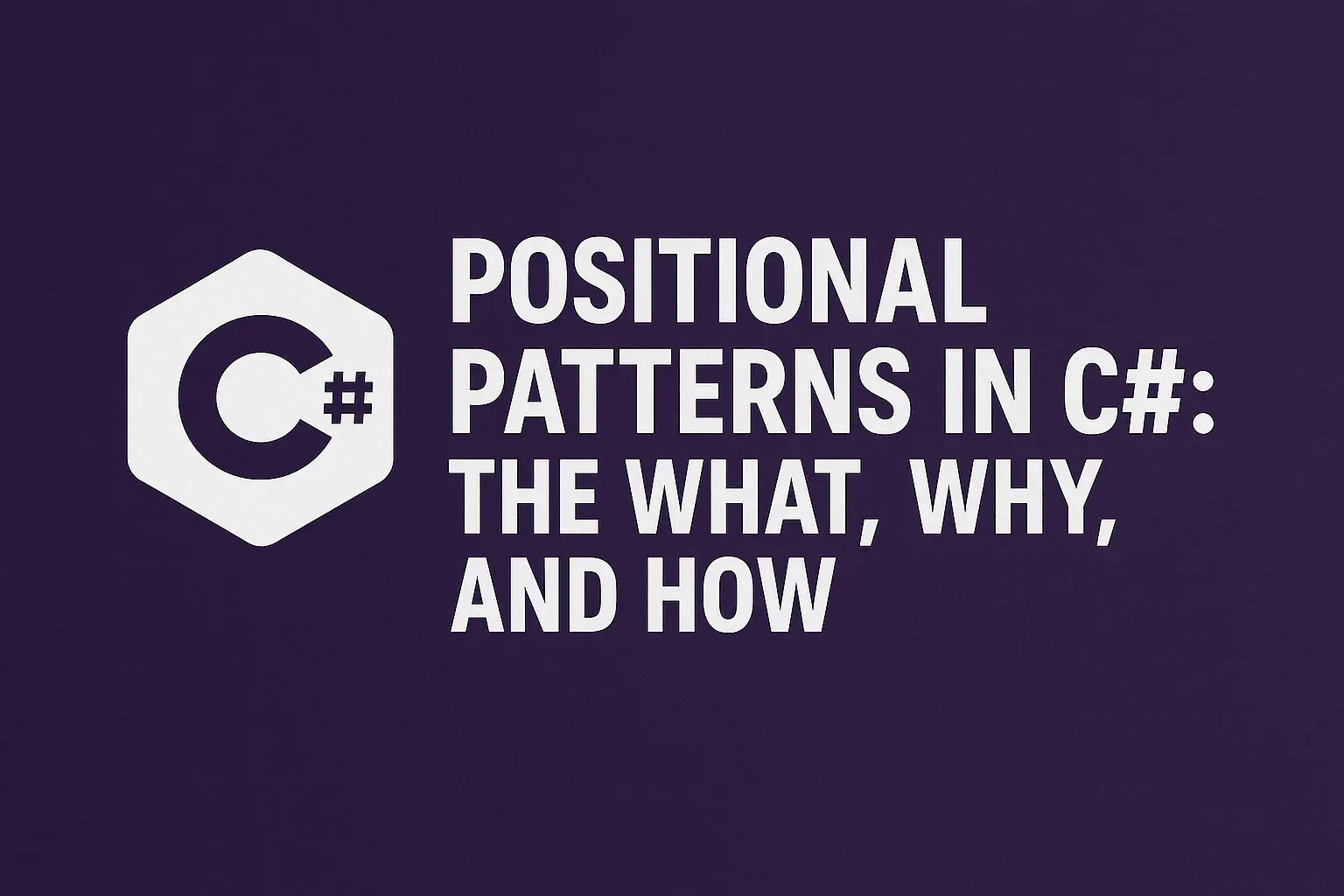
π What Are Positional Patterns?
Positional patterns allow you to deconstruct an object and match its components based on position (not property names).
They’re super handy for checking specific values or shapes of objects in a concise way.
π§ But Here’s the Catch:
You must define a Deconstruct()
method in your class or struct.
This method tells the compiler how to break your object down into pieces (positions).
π§ͺ Example: Matching a Point on the Axis
public class Point
{
public int X { get; }
public int Y { get; }
public Point(int x, int y) => (X, Y) = (x, y);
public void Deconstruct(out int x, out int y)
{
x = X;
y = Y;
}
}
Now we can use positional patterns:
var point = new Point(0, 5);
if (point is (0, _))
Console.WriteLine("Point lies on the Y axis");
else if (point is (_, 0))
Console.WriteLine("Point lies on the X axis");
Here, (0, _)
and (_, 0)
are positional patterns. Youβre matching the shape of the Point
object.
π With switch Statement
switch (point)
{
case (0, 0):
Console.WriteLine("Origin");
break;
case (0, _):
Console.WriteLine("Y Axis");
break;
case (_, 0):
Console.WriteLine("X Axis");
break;
default:
Console.WriteLine("Somewhere else");
break;
}
β What If You Don’t Have a Deconstruct Method?
If Deconstruct()
is not defined, you’ll get an error like:
CS8129: No suitable ‘Deconstruct’ instance or extension method was found…
But don’t worry β you can use property patterns instead!
β Property Pattern Alternative
public class Point
{
public int X { get; set; }
public int Y { get; set; }
}
if (point is { X: 0, Y: 0 })
Console.WriteLine("Origin");
Much cleaner than nested if
conditions, and no Deconstruct needed.
π Positional vs Property Patterns
Pattern Type | Needs Deconstruct() | Syntax Example |
---|---|---|
Positional Pattern | β Yes | is (0, _) |
Property Pattern | β No | is { X: 0, Y: _ } |
Both are useful β choose based on your use case.
π§ Summary
- Positional patterns are great for short, clean matching logic.
- You need to implement a
Deconstruct()
method. - If thatβs not feasible, property patterns are your friend.
- Combine with
switch
for readable, declarative matching.
C# pattern matching just keeps getting better, and positional patterns make it easier to write expressive and elegant code.
Try adding a Deconstruct()
method to your classes and see how it changes your matching logic.
Have you tried using positional patterns in your code yet? If not, give it a shot in your next project! β¨